The vast majority of web and mobile projects enable the viewing and manipulation of data. This usually requires an intense development effort in order to provide interaction with users. Due to number of different form factors that users can choose to interact with applications these days i.e. Laptop, Mobile, Tablets and wearable devices, brining simplicity and usability is a huge challenge. We also need to rely on frameworks to allow us to achieve this mission with the least amount of code and pain possible.
AngularJS offers a complete set of technologies that are capable of accomplishing any presentation, transformation, synchronization and validation of data in the UI, this is commonly referred to as Data Handling . AngularJS provides a very simple data handling syntax that radically shortens the learning curve.
In this tutorial we'll start refactoring the application we started developing in the first tutorial Angularjs Architectural Concepts and you will be focusing on 3 different data handling features in angular.
- Expressions
- Filters
- Form Validation
Expressions
An expression is a simple piece of code that will be evaluated by the framework and can be written between double curly brackets, i.e. {{ task.summary }}. This is termed as interpolation and allows you to easily interact with anything from the scope.
In the example above, for each iteration of the ngRepeat directive, a new child scope is created, which defines the boundaries of the expression. Expressions also forgive the undefined and null values, without displaying any error, instead it doesn't show anything.
Filters
filters enable us to manipulate and transform any value. Filters are useful when we need to format data such as Dates or currency to specific locales, or even to support the filtering of data in a grid component.
To make filters interact with the expression, we just need to put them inside double curly brackets:
Filters can be combined to create a chain where the output of filter1 is the input of filter2. Similar to the pipeline that exists in BASH
Standard Filters
The angular framework already brings with it a set of ready-to-use filters that are extremely useful in your daily development activities.
Currency
The currency filter is used to format a number based on a currency. The basic usage of the filter is without any parameter:
This will result in the display of the number as $100.00, formatted and prefixed with a dollar sign. This works great if you're working on site that based in the USA, but what about if the site you're working on needs to display values in Australian Dollars or any other currency. Fortunately its areas like this that the power of angular framework and the wider community helps out.
It is entirely possible to write your own custom currency filter which extends the capability or even replaces the standard currency filter. Check out this great tutorial
Angular.js, Accounting.js and Currency - How to Make Custom Filters in Angular.js
Date
The date filter is probably the most widely used filters. In most applications a date value will come from a database or any other source in a generic format, and will need to be transformed to meaningful format for users.
The output of the above may look something like this May 16, 2016 . There is often a requirement in applications to format a value using some optional mask:
When you use this format, the output changes to May 16/05/2016 09:42:10 .
In our Example application we're going to make some changes in order to illustrate how this works. Firstly we'll update our Tasks Json to include a Date Object, for kicks we're going input the dates as American format but we're going to want to display the Dates in UK Format on our webpage. Additionally we're going to enable the input of dates using the HTML5 Date input.
We're alson going to change the done in our JSON to be a boolean value and display it making use of a check box value.
We'll make a small modifications as below.
No when we run our Webpage we're going to see the following result.
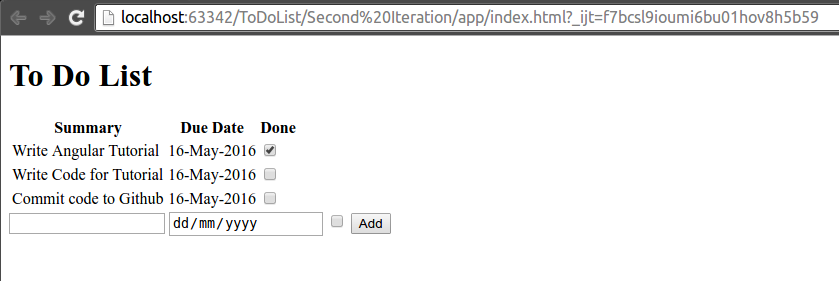
OrderBy
The orderBy filter, we can order any array based in a predicate expression. This expression is used to determin the order of the elements and works in three different ways:
[ebs_list] [ebs_li type="glyphicon-ok"]String: This is the property name. Also, there is an option to prefix+ or – to indicate the order direction. At the end of the day, +plate or
-plate are predicate expressions that will sort the array in an ascending
or descending order.[/ebs_li] [ebs_li type="glyphicon-ok"]Array: Based on the same concept of String's predicate expression, more than
one property can be added inside the array. Therefore, if two elements are
considered equivalent by the first predicate, the next one can be used, and
so on.[/ebs_li] [ebs_li type="glyphicon-ok"]Function: This function receives each element of the array as a parameter
and returns a number that will be used to compare the elements against
each other.[/ebs_li] [/ebs_list]
- String: This is the property name. Also, there is an option to prefix + or – to indicate the order direction. At the end of the day, +plate or -plate are predicate expressions that will sort the array in an ascending or descending order.
- Array: Based on the same concept of String's predicate expression, more than one property can be added inside the array. Therefore, if two elements are considered equivalent by the first predicate, the next one can be used, and so on.
- Function: This function receives each element of the array as a parameter and returns a number that will be used to compare the elements against each other.
In the following code, the orderBy filter is applied to an expression with the
predicate and reverse parameters:
In our To Do list application lets enable the user to sort the Done tasks. We're going to make some really simple changes. In order Table header we're going to insert a hyperlink for the Done and add ng-click attribute
We'll also add the orderBy filter on the ng-repeat
This will be enough to enable the sorting of done tasks. Our complete code should now look like the below. I have added some additional tasks.
There are a few other basic filters that come included with the angular framework and I won't be explaining them all in depth in the tutorial as some my merit a deeper discussion, and they are not entirely relevant to our little contrived example. These filters include;
- json
- limitTo
- lowercase
- number
- upercase
Form Validation
Almost every application has forms. It allows the users to type data that will be sent and processed at the backend. AngularJS provides a complete infrastructure to easily
create forms with the validation support.
The form will always be synchronized to its model with the two-way data binding mechanism, through the ngModel directive; therefore, the code is not required to
fulfill this purpose.
In our applicaiton we have enabled the user to add any tasks in but we haven't ensured that the have entered the basic data we need.The eager ones amongst might have already realises the we don't handle empty tasks, and if you click the add buttons twice when all fields are empty the applicaiton stops working. Lets fix this by adding some form validation. We're going to change our code slightly and introduce a form with and decorate the form with some additional attributes.
The complete source will now look a little like this
The validation here makes use of the standard HTML 5 validation, which will suffice for our example for now. We will be further expanding on this as we progress.
Our Application is still not ready for the prime time, but as you can see just a few simple code changes and we've improved it a little.
- What is this Directory.Packages.props file all about? - January 25, 2024
- How to add Tailwind CSS to Blazor website - November 20, 2023
- How to deploy a Blazor site to Netlify - November 17, 2023